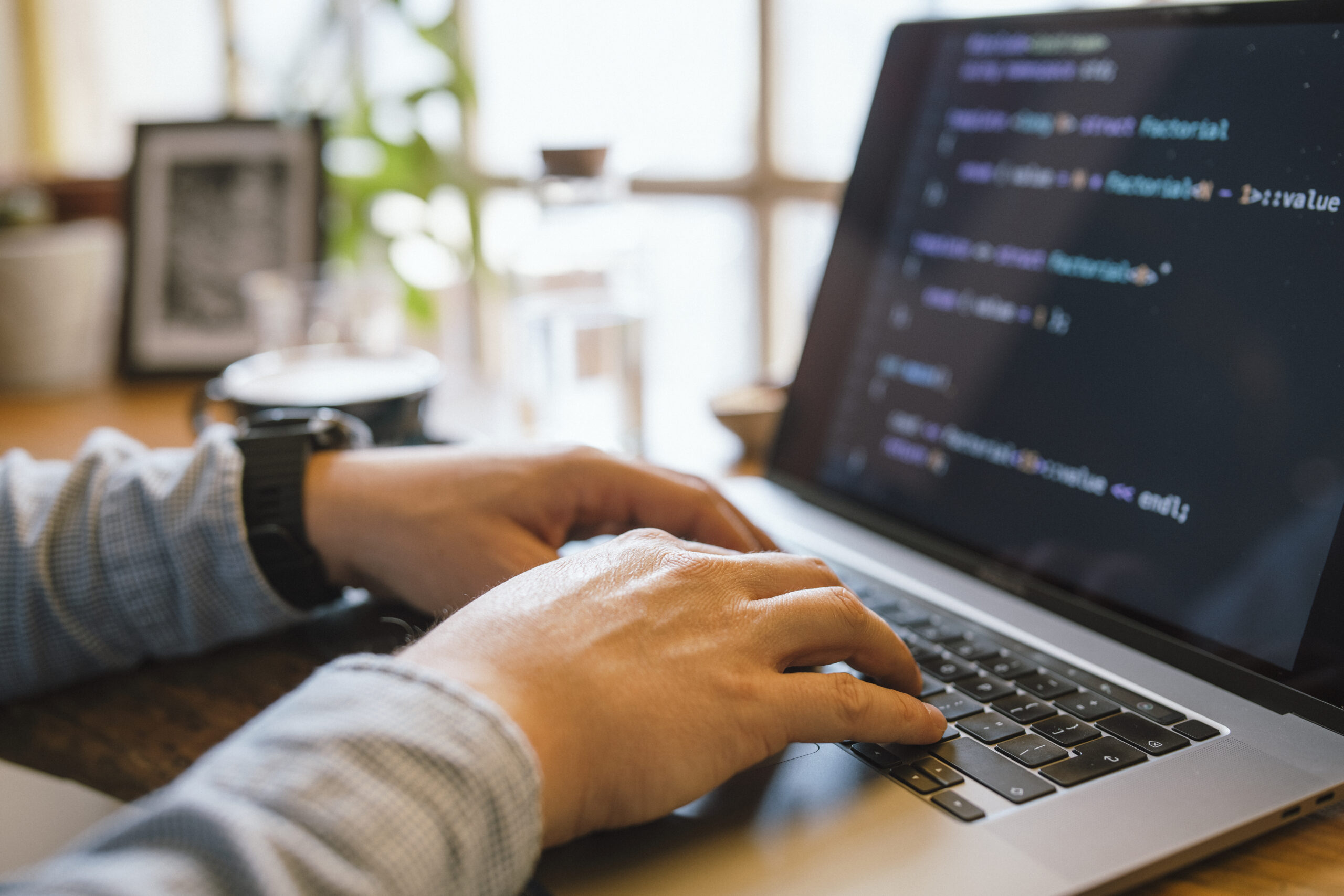
Debugging is Just about the most critical — however typically forgotten — skills inside a developer’s toolkit. It is not pretty much correcting damaged code; it’s about comprehending how and why items go Improper, and Finding out to think methodically to solve issues effectively. Regardless of whether you're a newbie or maybe a seasoned developer, sharpening your debugging competencies can help you save several hours of irritation and radically help your productivity. Here are several strategies to help builders stage up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods developers can elevate their debugging skills is by mastering the tools they use everyday. When composing code is a single A part of development, realizing how you can connect with it proficiently for the duration of execution is Similarly vital. Present day improvement environments come Geared up with strong debugging capabilities — but many developers only scratch the surface of what these instruments can do.
Get, as an example, an Built-in Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications assist you to established breakpoints, inspect the worth of variables at runtime, move by way of code line by line, and also modify code on the fly. When applied appropriately, they Permit you to observe specifically how your code behaves during execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for front-close developers. They help you inspect the DOM, check community requests, see real-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, sources, and community tabs can flip discouraging UI issues into workable tasks.
For backend or technique-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control about running processes and memory management. Mastering these tools might have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become cozy with Model Regulate techniques like Git to be aware of code record, find the exact moment bugs had been launched, and isolate problematic variations.
Ultimately, mastering your resources implies heading over and above default options and shortcuts — it’s about acquiring an personal knowledge of your improvement atmosphere in order that when concerns come up, you’re not shed at the hours of darkness. The greater you know your applications, the greater time you could expend solving the actual trouble rather than fumbling through the procedure.
Reproduce the situation
Among the most critical — and often missed — ways in productive debugging is reproducing the situation. Ahead of jumping to the code or producing guesses, developers have to have to produce a regular surroundings or scenario in which the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a sport of prospect, generally bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Request questions like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact ailments underneath which the bug occurs.
As you’ve collected more than enough details, seek to recreate the challenge in your local ecosystem. This might necessarily mean inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The difficulty appears intermittently, take into consideration creating automatic checks that replicate the edge situations or condition transitions included. These tests not merely enable expose the issue and also reduce regressions Sooner or later.
Sometimes, the issue can be environment-certain — it would materialize only on particular functioning systems, browsers, or below unique configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a move — it’s a attitude. It calls for endurance, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to correcting it. With a reproducible circumstance, You should utilize your debugging applications extra effectively, test potential fixes safely, and converse additional Plainly with the staff or people. It turns an summary grievance into a concrete problem — and that’s in which developers thrive.
Read and Understand the Mistake Messages
Mistake messages are often the most valuable clues a developer has when a little something goes Completely wrong. In lieu of observing them as annoying interruptions, developers should find out to treat mistake messages as immediate communications from your method. They often show you what precisely transpired, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the message thoroughly and in full. Quite a few developers, especially when underneath time strain, glance at the main line and promptly commence making assumptions. But further within the mistake stack or logs might lie the legitimate root lead to. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them very first.
Crack the error down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or functionality induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java typically abide by predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging method.
Some glitches are imprecise or generic, and in People conditions, it’s essential to examine the context wherein the error transpired. Look at associated log entries, input values, and up to date variations in the codebase.
Don’t forget about compiler or linter warnings both. These frequently precede more substantial difficulties and supply hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them accurately turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is Just about the most highly effective applications inside of a developer’s debugging toolkit. When used effectively, it provides real-time insights into how an application behaves, aiding you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging method begins with being aware of what to log and at what degree. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic information and facts all through progress, Details for standard functions (like profitable commence-ups), WARN for possible concerns that don’t break the application, Mistake for true difficulties, and FATAL in the event the technique can’t proceed.
Keep away from flooding your logs with extreme or irrelevant data. An excessive amount logging can obscure critical messages and slow down your system. Focus on critical functions, condition modifications, enter/output values, and demanding choice details within your code.
Format your log messages Plainly and persistently. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
During debugging, logs Permit you to monitor how variables evolve, what conditions are satisfied, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. Which has a effectively-thought-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and Enhance the Over-all maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not merely a technical activity—it is a method of investigation. To efficiently establish and take care of bugs, developers should technique the method similar to a detective resolving a secret. This state of click here mind can help stop working complex problems into manageable elements and comply with clues logically to uncover the basis bring about.
Get started by gathering evidence. Consider the indicators of the challenge: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, obtain just as much related info as you'll be able to without having jumping to conclusions. Use logs, check instances, and user reports to piece together a transparent photograph of what’s happening.
Next, variety hypotheses. Talk to you: What can be resulting in this habits? Have any alterations not too long ago been designed into the codebase? Has this issue happened right before underneath equivalent situations? The goal should be to slender down opportunities and establish likely culprits.
Then, examination your theories systematically. Attempt to recreate the problem inside of a managed surroundings. In the event you suspect a specific purpose or element, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcomes guide you closer to the reality.
Shell out near interest to compact information. Bugs frequently disguise while in the least expected sites—just like a lacking semicolon, an off-by-one mistake, or perhaps a race affliction. Be thorough and affected individual, resisting the urge to patch The problem without the need of completely understanding it. Short term fixes may disguise the real dilemma, just for it to resurface later.
And lastly, maintain notes on That which you tried and realized. Equally as detectives log their investigations, documenting your debugging process can help you save time for potential difficulties and help Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become simpler at uncovering concealed issues in complicated programs.
Generate Tests
Creating exams is among the best tips on how to boost your debugging techniques and In general development efficiency. Exams not merely support capture bugs early and also function a security Web that gives you self-assurance when producing alterations on your codebase. A very well-analyzed software is much easier to debug mainly because it helps you to pinpoint accurately where and when a difficulty happens.
Begin with unit tests, which concentrate on person functions or modules. These little, isolated tests can quickly expose irrespective of whether a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know the place to search, substantially decreasing the time used debugging. Device checks are In particular valuable for catching regression bugs—concerns that reappear right after previously remaining fastened.
Following, integrate integration checks and close-to-conclude checks into your workflow. These support make sure that various portions of your application work alongside one another efficiently. They’re especially practical for catching bugs that come about in sophisticated systems with many elements or services interacting. If a thing breaks, your exams can show you which Portion of the pipeline unsuccessful and beneath what circumstances.
Producing exams also forces you to definitely Feel critically about your code. To check a function thoroughly, you will need to understand its inputs, anticipated outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and less bugs.
When debugging a difficulty, creating a failing exam that reproduces the bug may be a strong starting point. After the take a look at fails consistently, it is possible to deal with fixing the bug and look at your exam move when The difficulty is resolved. This strategy makes sure that the same bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a annoying guessing activity right into a structured and predictable approach—encouraging you capture much more bugs, more rapidly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s effortless to be immersed in the situation—gazing your screen for hours, attempting Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen stress, and sometimes see The problem from a new standpoint.
If you're much too near the code for far too extensive, cognitive exhaustion sets in. You would possibly get started overlooking noticeable glitches or misreading code you wrote just hrs previously. In this particular condition, your Mind gets significantly less productive at difficulty-solving. A brief wander, a coffee break, or even switching to a different endeavor for ten–15 minutes can refresh your concentrate. A lot of developers report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also assist reduce burnout, In particular for the duration of for a longer time debugging sessions. Sitting before a display, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you right before.
In case you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to move all around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a sensible technique. It offers your Mind space to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight That always blocks your progress. Debugging can be a psychological puzzle, and rest is part of fixing it.
Study From Every Bug
Each individual bug you encounter is much more than simply A short lived setback—it's an opportunity to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you one thing worthwhile when you take some time to mirror and assess what went Completely wrong.
Begin by asking oneself a number of critical thoughts as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit testing, code opinions, or logging? The responses normally expose blind places as part of your workflow or knowledge and assist you Establish much better coding behaviors transferring ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see styles—recurring troubles or frequent errors—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Specifically potent. Irrespective of whether it’s through a Slack concept, a short generate-up, or A fast information-sharing session, helping Many others stay away from the same difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your mentality from disappointment to curiosity. Instead of dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, a few of the finest developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, each bug you correct provides a fresh layer towards your talent established. So up coming time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, much more able developer because of it.
Conclusion
Strengthening your debugging skills will take time, exercise, and patience — nevertheless the payoff is large. It makes you a more productive, self-assured, and able developer. The following time you happen to be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be better at Everything you do.